Understanding PHP Sockets and the WebSocket API in JavaScript
Understanding PHP Sockets and the WebSocket API in JavaScript
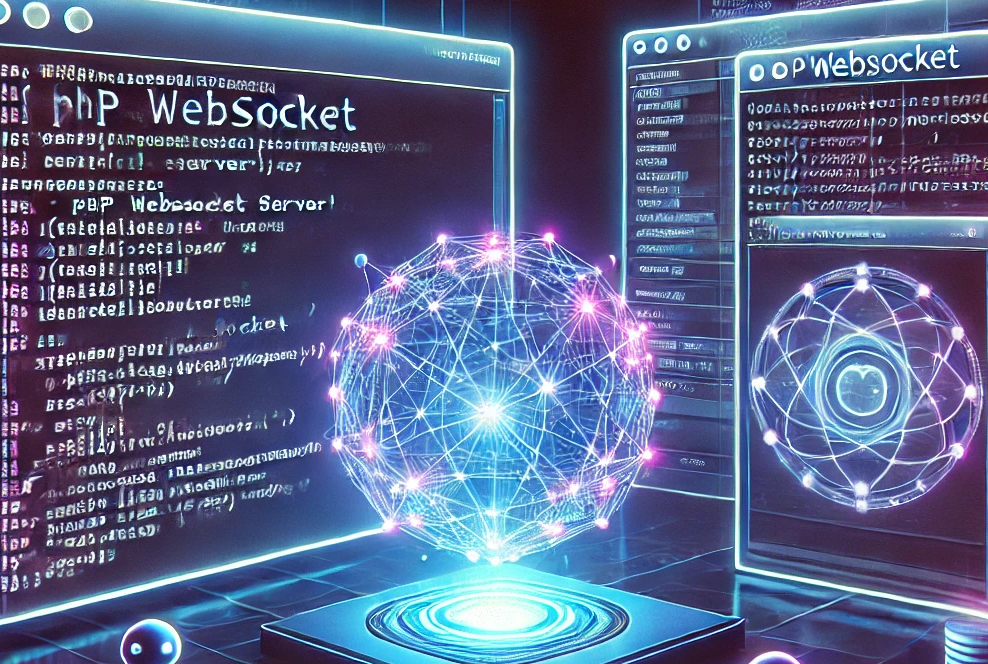
Understanding PHP Sockets and the WebSocket API in JavaScript
WebSockets are essential for real-time web applications, enabling persistent, full-duplex communication between a client and a server. While PHP is not typically known for real-time applications, it provides socket functionalities that allow developers to create WebSocket servers. In this blog, we will explore PHP sockets, the WebSocket API in JavaScript, and how a simple PHP-based WebSocket server can be implemented using a reference package.
Why Use WebSockets?
Unlike traditional HTTP, which follows a request-response model, WebSockets allow bidirectional communication without repeatedly polling the server. This is useful for applications like:
- Chat applications
- Real-time notifications
- Collaborative tools
- Live data streaming (stock prices, sports scores, etc.)
Understanding PHP Sockets
PHP provides a set of functions to create and manage sockets, including:
socket_create()
: Creates a new socket.socket_bind()
: Binds the socket to an IP and port.socket_listen()
: Listens for incoming connections.socket_accept()
: Accepts a client connection.socket_read()
: Reads data from a socket.socket_write()
: Sends data to a socket.socket_close()
: Closes a socket connection.
These functions allow PHP to establish low-level TCP connections, which can be extended to support the WebSocket protocol.
The WebSocket API in JavaScript
The WebSocket API provides a simple way to interact with WebSocket servers using JavaScript. A WebSocket connection can be established as follows:
This JavaScript code connects to a WebSocket server, sends a message upon connection, and listens for incoming messages.
Building a WebSocket Server in PHP
To establish a WebSocket server in PHP, we need to:
- Set up a socket server.
- Perform a WebSocket handshake.
- Handle client messages.
- Send messages back to clients.
Example PHP WebSocket Server
Below is a basic PHP WebSocket server:
Using the Provided PHP WebSocket Code
If you are looking for a more complete implementation, the PHP WebSocket package included in this blog provides a working example of:
- A WebSocket server in PHP.
- A JavaScript client using the WebSocket API.
- Proper WebSocket handshake handling.
- Broadcasting messages to multiple clients.
By studying this package, you can:
- Extend it for real-time chat applications.
- Implement real-time notifications.
- Learn how PHP sockets work with WebSockets.
Clone this reference code from here
WebSockets in PHP might not be as common as in Node.js, but they can be powerful for real-time communication when implemented correctly. With proper understanding of PHP sockets and the WebSocket API in JavaScript, you can create efficient real-time applications. The provided package serves as an excellent reference to get started.